Next.js
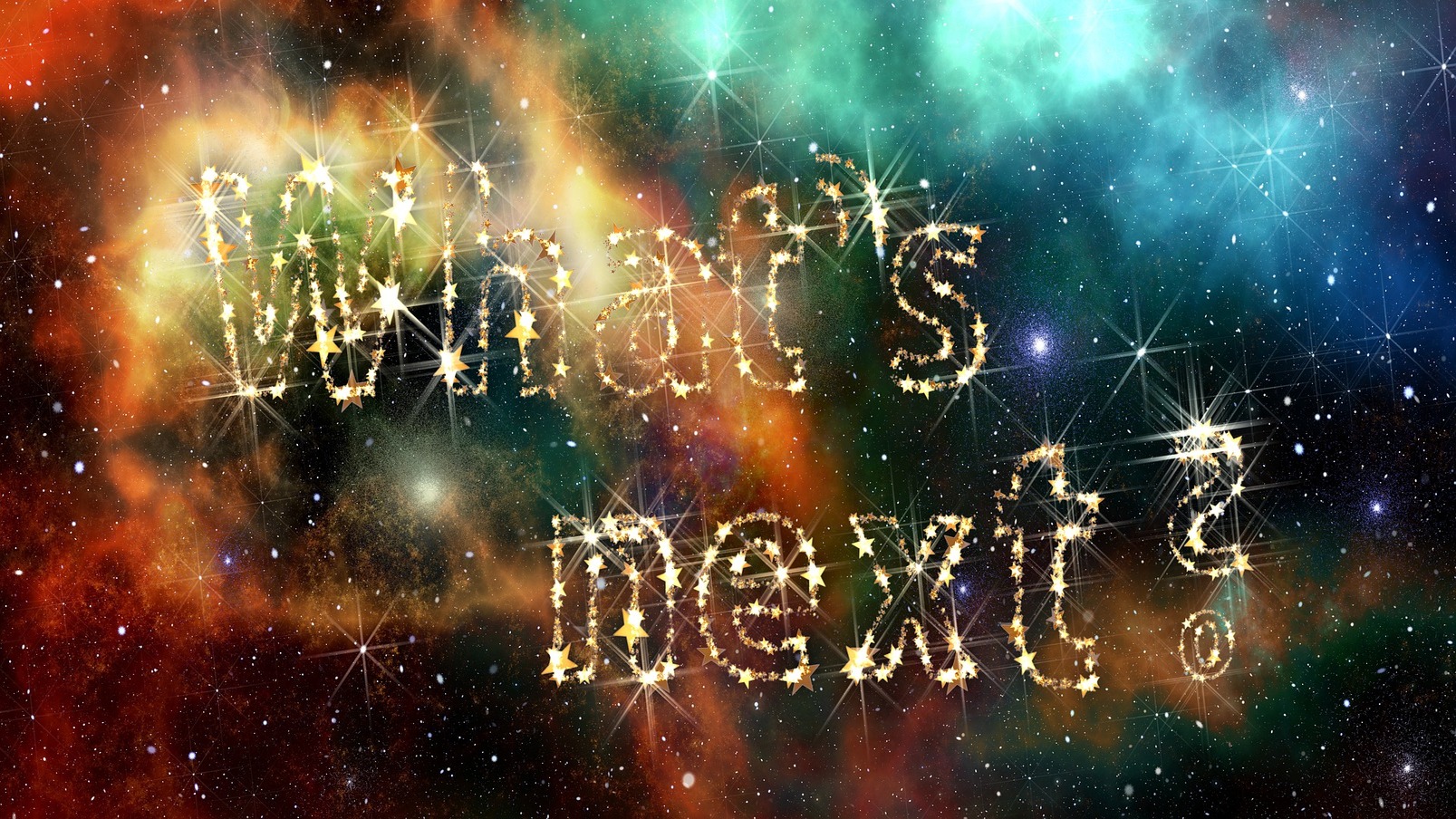
1. What is Next.js?
The React Framework for the full-stack Web applications
- Full Stack
- File-based routing
- SEO, Image, Font Optimization
- Server Side Rendering (+Hybrid Rendering)
1.1 React
A javascript library for building user interfaces.
- SPA (Single Page Application)
- CSR (Client Side Rendering)
Libraries
- A tool that can be used to select only what is needed to solve a specific problem.
Frameworks
- Provides a solution for larger units rather than solving specific (small unit) problems.
- We can implement our logic within the designated framework.
1.2 History of Next.js
OCT 2016
Vercel, which provides a cloud platform as a service (PaaS1), has released its first release.
6 Principles
- out-of-the-box functionality requiring no setup
- JavaScript everywhere(Frontned & Backend -> Full Stack)
- automatic code-splitting2 and server-rendering
- configurable data-fetching
- anticipating requests
- simplifying deployment
JULY 2020
- Rewrite, Redirect
- Incremental Static Generation
OCT 2022 (Next.js 13)
- new routing (/app)
- (nested) layouts
- Server Components
- streaming
- new toolchain (Turbopack)
1.3 CSR
Cliend Side Rendering
- The entity that does the rendering is the client (browser).
Process
- Browser requests the webpage.
- Web server sends basic HTML, CSS, and JavaScript files to the client, which includes the React application code.
- Browser renders HTML and CSS and then loads and executes JavaScript.
- JavaScript initializes the React application.
- React creates a DOM and renders it on the screen. React generates a virtual DOM based on the state and components of the webpage and updates the actual DOM incrementally.
- As the application’s state changes due to user interaction, React regenerates the virtual DOM and updates only the required parts of the actual DOM, thus enhancing performance.
- If the application requires additional data, it communicates with the server via Ajax3 to fetch the data and update accordingly. Throughout this process, React provides a fast and sophisticated user experience.
Advantages
- Once loaded, it provides a fast UX
- Fetches data partially through Ajax and updates partially
- Low server load
Disadvantages
- Takes longer to load the page (TTV4/FCP5)
- JavaScript activation is necessary
- SEO optimization is challenging
- Vulnerable to security issues
- Caching on CDN6 is not available
⬇️To resolve these issues!⬇️
1.4 SSG
Static Site Generation
- The entity that does the rendering is the server.
- Rendering at build time.
Process
- The user requests a specific page from their web browser.
- The hosting platform server sends the pre-built static HTML, CSS, and JavaScript files associated with the requested page to the user’s browser. The browser does not need to make additional requests to the server for dynamic data as the content is pre-rendered during the build process.
- The browser displays the initially rendered HTML and CSS content of the page. At this point, the user can see the page content. The JavaScript associated with the page, containing the React application code and any hydration7 logic, also begins loading and executing during this step.
- When the JavaScript finishes loading and executing, the browser hydrates the necessary parts of the page. Hydration involves attaching the JavaScript event handlers and other interactive functionality to the static HTML content.
- Once hydrated, the page provides full interactivity. If a part of the page needs to fetch additional data or update the content through user interactions or events, the application typically uses client-side data fetching (like fetch or a library such as Axios) to communicate with an API and update the content.
Advantages
- Fast page loading time (TTV/FCP)
- JavaScript is not required
- Good SEO optimization
- Excellent security
- Cached on CDN
Disadvantages
- Data is static
- Difficult to provide user-specific information
⬇️To resolve these issues!⬇️
1.5 ISR
Incremental Static Regeneration
- The entity that does the rendering is the server.
- Rendering at build time, then rendering periodically
Advantages
- Fast page loading time (TTV/FCP)
- JavaScript is not required
- Good SEO optimization
- Excellent security
- Cached on CDN
- Data is updated periodically
Disadvantages
- Still not real-time data
- Still challenging to provide user-specific information
⬇️To resolve these issues!⬇️
1.6 SSR
Server Side Rendering
- The entity that does the rendering is the server.
- Rendering at run time, upon request
Advantages
- Fast page loading time (TTV/FCP)
- JavaScript is not required
- Good SEO optimization
- Excellent security
- Uses real-time data
- Utilizes user-specific data
Disadvantages
- Can be relatively slow
- Can put a strain on the server
- Caching on CDN is not available
Process
- The user requests a specific page from their web browser.
- The server receives the request and runs the getServerSideProps function, which fetches data or performs any necessary server-side computations. It then generates the final HTML, CSS, and JavaScript files, including the fetched data, and sends these files to the user’s browser.
- The browser displays the rendered HTML and CSS content of the page. At this point, users can see the page content. Concurrently, the browser loads and executes the JavaScript associated with the page, containing the React application code and any hydration logic.
- After the JavaScript finishes loading and executing, the browser hydrates the necessary parts of the page. Hydration involves attaching JavaScript event handlers and other interactive functionality to the server-rendered HTML content.
- Once hydrated, the page provides full interactivity. If a part of the page needs to fetch additional data or update content through user interactions or events, the application typically uses client-side data fetching (like fetch or a library such as Axios) to communicate with an API and update the content.
Hybrid! : To build a high-performance, powerful web app, use a combination of CSR, SSG, ISR, and SSR!
1.7 Important points in web app development
Reducing TTV (Time To View)!
Reducing TTI (Time To Interact)!
It’s important to narrow the gap between the static HTML page and the hydrated page! (The gap from the moment you view the page to when you can use it) Alternatively, if this gap can’t be reduced, it is important how clearly you can inform the user about this gap!
1.8 Next.js Decision Tree
- User? (Is user login required? Is user-specific data different?)
- Static? (Does data change?)
- Often? (Does data change frequently?)
- 1 -> NO, 2 -> NO: SSG (For stateless pages, Next.js defaults to SSG)
- 1 -> NO, 2 -> YES, 3 -> NO: ISR
- 1 -> NO, 2 -> YES, 3 -> YES: SSR/Hybrid (ISR|SSG + CSR)
- 1 -> YES: CSR/SSR/Hybrid (SSG+CSR)
A service that automatically manages the OS, hardware specs, etc., required to operate a service once it is deployed. ↩︎
Instead of sending the entire bundled code to the user, it sends small pieces of the code corresponding to the part the user is viewing. (small -> fast) ↩︎
Asynchronous JavaScript and XML, is a web development technique that allows web applications to communicate with a server and exchange data in the background. This enables the web application to update or modify its content without having to completely reload the page. Ajax works by using JavaScript to make asynchronous HTTP requests to a server, process the server’s response (usually in XML or JSON format), and then update the web page’s content using DOM manipulation. This results in a more responsive and interactive user experience because only the necessary data is exchanged with the server, and the whole page isn’t reloaded each time a user interacts with the web application. In modern web development, Ajax has become a standard technique, and various libraries and frameworks like jQuery, Angular, and React have built-in utilities for managing Ajax requests. Though the name implies the use of XML, JSON has become the preferred data format for many Ajax implementations, as it is more lightweight and easier to work with in JavaScript. ↩︎
Time To View ↩︎
First Contentful Paint ↩︎
Content Delievery Network ↩︎
Hydration for interaction. Stay Hydrated. Filling with water (React). Next.js first sends the pre-rendered static HTML page (not yet containing JavaScript -> no response when clicked) to the client so that users can see it quickly. After that, JavaScript and React code are sent to the client, and when the download is completed on the client side, the page is filled with React. In other words, component rendering takes place. -> It becomes possible to handle clicks. ↩︎