Set
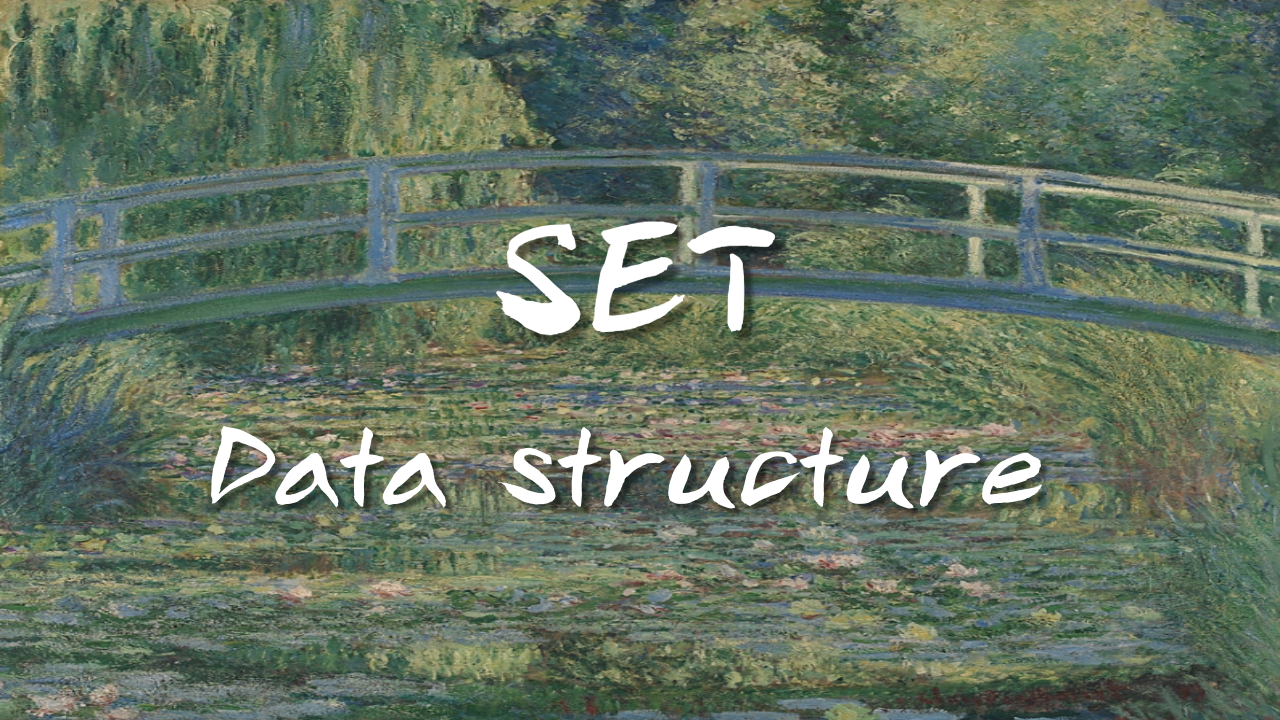
La Seine a Argenteuil, 1875, Claude Monet
1. What is Set?
The set data structure is kind of like an array except there are no duplicate. items and the values are not in any particular order.
The typical use for set is to simply check for the presence of an item.
2. Set Implementation
// title: 'Set.js'
// Why it's named this way is because we want to make it distinct from the es5.
class mySet {
constructor() {
// collection will hold the set
this.collection = [];
}
// has method will check for the presence of an element and return true or false
has(element) {
return (this.collection.indexOf(element) !== -1);
}
// values method will return all the values in the set
values(){
return this.collection;
}
// add method will add an element to the set
add(element) {
if(!this.has(element)){
this.collection.push(element);
return this.collection;
}
return this.collection;
}
// delete method will remove an element from a set
delete(element) {
if(this.has(element)){
index = this.collection.indexOf(element);
this.collection.splice(index,1);
return true;
}
return false;
}
// size method will return the size of the collection
// In the es6 'size' is just property. (You're not going to put parenthesis at after the the method)
size() {
return this.collection.length;
}
// union method will return the union of two sets
union(otherSet) {
let unionSet = new mySet();
let firstSet = this.values();
let secondSet = otherSet.values();
firstSet.forEach(function(e){
unionSet.add(e)
})
secondSet.forEach(function(e){
unionSet.add(e)
})
return unionSet;
}
// intersection method will return the intersection of two sets as a new set
intersection(otherSet) {
let intersectionSet = new mySet();
let firstSet = this.values();
firstSet.forEach(function(e){
if(otherSet.has(e)){
intersectionSet.add(e)
}
})
return intersectionSet
}
// difference method will return the difference of two sets as a new set
difference(otherSet) {
let differenceSet = new mySet();
let firstSet = this.values();
firstSet.forEach(function(e){
if(!otherSet.has(e)){
differenceSet.add(e);
}
})
return differenceSet;
}
// isSuperset method will test if the set is a subset of a difference set
isSuperset(otherSet){
let firstSet = this.values();
return firstSet.every(function(value){
return otherSet.has(value);
})
}
}
//[[Prototype]]: Array
let setA = new mySet();
let setB = new mySet();
setA.add('a');
setB.add('b');
setB.add('c');
setB.add('a');
setB.add('d');
console.log(setA.add('d')) // ['a','d']
console.log(setA.isSuperset(setB)) // true
console.log(setA.intersection(setB).values()) // ['a','d']
console.log(setB.difference(setA).values()) // ['b','c']
// In es6 [[Prototype]]: Set
let setC = new Set();
let setD = new Set();
setC.add('a');
setD.add('b');
setD.add('c');
setD.add('a');
setD.add('d');
console.log(setD.values()) // {'b','c','a','d'}
setD.delete('a')
console.log(setD.has('a')) // false
console.log(setD.add('d')) // {'b','c','d'}
https://www.freecodecamp.org/
https://www.codesdope.com/
https://algorithmtutor.com/
https://blog.penjee.com/learnprogramming/programming-gifs/
https://dev.to/abdisalan_js/4-ways-to-traverse-binary-trees-with-animations-5bi5
https://www.programiz.com/